在Spring Boot中,實現Excel表格導出的方式有很多種,以下是幾種常見的方法:
- 使用Apache POI:Apache POI是一個開源的Java API,用于處理Microsoft Office文檔格式,包括Excel電子表格。在Spring Boot中,可以使用Apache POI創建Excel文檔,并將其寫入HTTP響應中,以實現Excel表格的導出。
- 使用EasyPOI:EasyPOI是一個開源的Java API,用于處理Excel電子表格。它基于Apache POI和Jxls開發,提供了更加簡單易用的API,可以幫助我們快速實現Excel表格的導出。
- 使用Jxls:Jxls是一個用于生成Excel報表的Java庫。在Spring Boot中,可以使用Jxls創建Excel文檔,并將其寫入HTTP響應中,以實現Excel表格的導出。
- 使用第三方庫:還有其他一些第三方的Java庫可以用于生成Excel電子表格,例如Aspose.Cells、JExcelApi等,它們也可以在Spring Boot中使用,實現Excel表格的導出。
需要注意的是,無論使用哪種方法,都需要將Excel文檔寫入HTTP響應中,并設置正確的Content-Type和Content-Disposition頭信息,以確保瀏覽器能夠正確地識別Excel文檔并下載它。文章源自四五設計網-http://www.wasochina.com/43669.html
一、Apache POI
- maven依賴坐標
1 2 3 4 5 6 7 8 9 10 | < dependency > ?? < groupId >org.apache.poi</ groupId > ?? < artifactId >poi</ artifactId > ?? < version >4.1.2</ version > </ dependency > < dependency > ?? < groupId >org.apache.poi</ groupId > ?? < artifactId >poi-ooxml</ artifactId > ?? < version >4.1.2</ version > </ dependency > |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | @RestController public class ExcelController { ?? @GetMapping ( "/export" ) ???? // 創建Excel文檔 ???? XSSFWorkbook workbook = new XSSFWorkbook(); ???? XSSFSheet sheet = workbook.createSheet( "Sheet1" ); ???? // 創建表頭 ???? XSSFRow header = sheet.createRow( 0 ); ???? header.createCell( 0 ).setCellValue( "姓名" ); ???? header.createCell( 1 ).setCellValue( "年齡" ); ???? header.createCell( 2 ).setCellValue( "性別" ); ???? // 填充數據 ???? List<User> users = getUserList(); ???? int rowIndex = 1 ; ???? for (User user : users) { ?????? XSSFRow row = sheet.createRow(rowIndex++); ?????? row.createCell( 0 ).setCellValue(user.getName()); ?????? row.createCell( 1 ).setCellValue(user.getAge()); ?????? row.createCell( 2 ).setCellValue(user.getGender()); ???? } ???? // 設置響應頭信息 ???? response.setContentType( "application/vnd.ms-excel" ); ???? response.setHeader( "Content-Disposition" , "attachment; filename=users.xlsx" ); ???? // 將Excel文檔寫入響應流中 ???? ServletOutputStream outputStream = response.getOutputStream(); ???? workbook.write(outputStream); ???? outputStream.flush(); ???? outputStream.close(); ?? } ?? // 模擬獲取用戶數據 ?? private List<User> getUserList() { ???? List<User> users = new ArrayList<>(); ???? users.add( new User( "張三" , 25 , "男" )); ???? users.add( new User( "李四" , 30 , "女" )); ???? users.add( new User( "王五" , 28 , "男" )); ???? return users; ?? } ?? // 用戶實體類 ?? private static class User { ???? private String name; ???? private int age; ???? private String gender; ???? public User(String name, int age, String gender) { ?????? this .name = name; ?????? this .age = age; ?????? this .gender = gender; ???? } ???? public String getName() { ?????? return name; ???? } ???? public void setName(String name) { ?????? this .name = name; ???? } ???? public int getAge() { ?????? return age; ???? } ???? public void setAge( int age) { ?????? this .age = age; ???? } ???? public String getGender() { ?????? return gender; ???? } ???? public void setGender(String gender) { ?????? this .gender = gender; ???? } ?? } } |
二、Easy POI
- maven依賴坐標
1 2 3 4 5 6 7 8 9 10 | < dependency > ?? < groupId >cn.afterturn</ groupId > ?? < artifactId >easypoi-base</ artifactId > ?? < version >4.2.0</ version > </ dependency > < dependency > ?? < groupId >cn.afterturn</ groupId > ?? < artifactId >easypoi-web</ artifactId > ?? < version >4.2.0</ version > </ dependency > |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | @RestController public class ExcelController { ?? @GetMapping ( "/export" ) ?? public void exportExcel(HttpServletResponse response) throws Exception { ???? // 創建Excel文檔 ???? Workbook workbook = ExcelExportUtil.exportExcel( new ExportParams( "用戶列表" , "用戶信息" ), User. class , getUserList()); ???? // 設置響應頭信息 ???? response.setContentType( "application/vnd.ms-excel" ); ???? response.setHeader( "Content-Disposition" , "attachment; filename=users.xlsx" ); ???? // 將Excel文檔寫入響應流中 ???? ServletOutputStream outputStream = response.getOutputStream(); ???? workbook.write(outputStream); ???? outputStream.flush(); ???? outputStream.close(); ?? } ?? // 模擬獲取用戶數據 ?? private List<User> getUserList() { ???? List<User> users = new ArrayList<>(); ???? users.add( new User( "張三" , 25 , "男" )); ???? users.add( new User( "李四" , 30 , "女" )); ???? users.add( new User( "王五" , 28 , "男" )); ???? return users; ?? } ?? // 用戶實體類 ?? private static class User { ???? @Excel (name = "姓名" , orderNum = "0" ) ???? private String name; ???? @Excel (name = "年齡" , orderNum = "1" ) ???? private int age; ???? @Excel (name = "性別" , orderNum = "2" ) ???? private String gender; ???? public User(String name, int age, String gender) { ?????? this .name = name; ?????? this .age = age; ?????? this .gender = gender; ???? } ???? public String getName() { ?????? return name; ???? } ???? public void setName(String name) { ?????? this .name = name; ???? } ???? public int getAge() { ?????? return age; ???? } ???? public void setAge( int age) { ?????? this .age = age; ???? } ???? public String getGender() { ?????? return gender; ???? } ???? public void setGender(String gender) { ?????? this .gender = gender; ???? } ?? } } |
三、Jxls
- maven依賴坐標
1 2 3 4 5 6 7 8 9 10 | < dependency > ?? < groupId >org.jxls</ groupId > ?? < artifactId >jxls</ artifactId > ?? < version >2.14.0</ version > </ dependency > < dependency > ?? < groupId >org.jxls</ groupId > ?? < artifactId >jxls-poi</ artifactId > ?? < version >2.14.0</ version > </ dependency > |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | @RestController public class ExcelController { ?? @GetMapping ( "/export" ) ?? public void exportExcel(HttpServletResponse response) throws Exception { ???? // 加載Excel模板 ???? InputStream inputStream = getClass().getResourceAsStream( "/templates/user_template.xlsx" ); ???? Workbook workbook = WorkbookFactory.create(inputStream); ???? // 填充數據 ???? List<User> users = getUserList(); ???? Map<String, Object> model = new HashMap<>(); ???? model.put( "users" , users); ???? JxlsHelper.getInstance().processTemplate(model, workbook.getSheetAt( 0 )); ???? // 設置響應頭信息 ???? response.setContentType( "application/vnd.ms-excel" ); ???? response.setHeader( "Content-Disposition" , "attachment; filename=users.xlsx" ); ???? // 將Excel文檔寫入響應流中 ???? ServletOutputStream outputStream = response.getOutputStream(); ???? workbook.write(outputStream); ???? outputStream.flush(); ???? outputStream.close(); ?? } ?? // 模擬獲取用戶數據 ?? private List<User> getUserList() { ???? List<User> users = new ArrayList<>(); ???? users.add( new User( "張三" , 25 , "男" )); ???? users.add( new User( "李四" , 30 , "女" )); ???? users.add( new User( "王五" , 28 , "男" )); ???? return users; ?? } ?? // 用戶實體類 ?? private static class User { ???? private String name; ???? private int age; ???? private String gender; ???? public User(String name, int age, String gender) { ?????? this .name = name; ?????? this .age = age; ?????? this .gender = gender; ???? } ???? public String getName() { ?????? return name; ???? } ???? public void setName(String name) { ?????? this .name = name; ???? } ???? public int getAge() { ?????? return age; ???? } ???? public void setAge( int age) { ?????? this .age = age; ???? } ???? public String getGender() { ?????? return gender; ???? } ???? public void setGender(String gender) { ?????? this .gender = gender; ???? } ?? } } |
總結
到此這篇關于springboot實現excel表格導出幾種常見方法的文章就介紹到這了文章源自四五設計網-http://www.wasochina.com/43669.html 文章源自四五設計網-http://www.wasochina.com/43669.html
繼續閱讀
我的微信
微信掃一掃
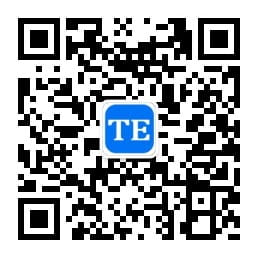
我的微信
惠生活福利社
微信掃一掃

我的公眾號
評論