在Unity中,要獲取Texture的內(nèi)存文件大小,可以使用UnityEditor.TextureUtil類中的一些函數(shù)。這些函數(shù)提供了獲取存儲(chǔ)內(nèi)存大小和運(yùn)行時(shí)內(nèi)存大小的方法。由于UnityEditor.TextureUtil是一個(gè)內(nèi)部類,我們需要使用反射來(lái)訪問它。
步驟
- 導(dǎo)入U(xiǎn)nityEditor命名空間和System.Reflection命名空間:
1 2 | using UnityEditor; using System.Reflection; |
- 創(chuàng)建一個(gè)函數(shù)來(lái)獲取Texture的內(nèi)存文件大?。?/li>
1 2 3 4 5 6 7 8 9 10 11 | public static long GetTextureFileSize(Texture2D texture) { ???? long fileSize = 0; ???? // 使用反射獲取UnityEditor.TextureUtil類的Type ???? Type textureUtilType = typeof (TextureImporter).Assembly.GetType( "UnityEditor.TextureUtil" ); ???? // 使用反射獲取UnityEditor.TextureUtil類的GetStorageMemorySizeLong方法 ???? MethodInfo getStorageMemorySizeLongMethod = textureUtilType.GetMethod( "GetStorageMemorySizeLong" , BindingFlags.Static | BindingFlags.Public); ???? // 調(diào)用GetStorageMemorySizeLong方法獲取存儲(chǔ)內(nèi)存大小 ???? fileSize = ( long )getStorageMemorySizeLongMethod.Invoke( null , new object [] { texture }); ???? return fileSize; } |
- 創(chuàng)建一個(gè)函數(shù)來(lái)獲取Texture的運(yùn)行時(shí)內(nèi)存大?。?/li>
1 2 3 4 5 6 7 8 9 10 11 | public static long GetTextureRuntimeMemorySize(Texture2D texture) { ???? long memorySize = 0; ???? // 使用反射獲取UnityEditor.TextureUtil類的Type ???? Type textureUtilType = typeof (TextureImporter).Assembly.GetType( "UnityEditor.TextureUtil" ); ???? // 使用反射獲取UnityEditor.TextureUtil類的GetRuntimeMemorySizeLong方法 ???? MethodInfo getRuntimeMemorySizeLongMethod = textureUtilType.GetMethod( "GetRuntimeMemorySizeLong" , BindingFlags.Static | BindingFlags.Public); ???? // 調(diào)用GetRuntimeMemorySizeLong方法獲取運(yùn)行時(shí)內(nèi)存大小 ???? memorySize = ( long )getRuntimeMemorySizeLongMethod.Invoke( null , new object [] { texture }); ???? return memorySize; } |
示例代碼
示例 1:獲取Texture的存儲(chǔ)內(nèi)存大小
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | using UnityEngine; using UnityEditor; using System.Reflection; public class TextureSizeExample : MonoBehaviour { ???? [SerializeField] ???? private Texture2D texture; ???? private void Start() ???? { ???????? long fileSize = GetTextureFileSize(texture); ???? } ???? private static long GetTextureFileSize(Texture2D texture) ???? { ???????? long fileSize = 0; ???????? Type textureUtilType = typeof (TextureImporter).Assembly.GetType( "UnityEditor.TextureUtil" ); ???????? MethodInfo getStorageMemorySizeLongMethod = textureUtilType.GetMethod( "GetStorageMemorySizeLong" , BindingFlags.Static | BindingFlags.Public); ???????? fileSize = ( long )getStorageMemorySizeLongMethod.Invoke( null , new object [] { texture }); ???????? return fileSize; ???? } } |
示例 2:獲取Texture的運(yùn)行時(shí)內(nèi)存大小
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | using UnityEngine; using UnityEditor; using System.Reflection; public class TextureSizeExample : MonoBehaviour { ???? [SerializeField] ???? private Texture2D texture; ???? private void Start() ???? { ???????? long memorySize = GetTextureRuntimeMemorySize(texture); ???????? Debug.Log( "Texture Runtime Memory Size: " + memorySize + " bytes" ); ???? } ???? private static long GetTextureRuntimeMemorySize(Texture2D texture) ???? { ???????? long memorySize = 0; ???????? Type textureUtilType = typeof (TextureImporter).Assembly.GetType( "UnityEditor.TextureUtil" ); ???????? MethodInfo getRuntimeMemorySizeLongMethod = textureUtilType.GetMethod( "GetRuntimeMemorySizeLong" , BindingFlags.Static | BindingFlags.Public); ???????? memorySize = ( long )getRuntimeMemorySizeLongMethod.Invoke( null , new object [] { texture }); ???????? return memorySize; ???? } } |
示例 3:同時(shí)獲取Texture的存儲(chǔ)內(nèi)存大小和運(yùn)行時(shí)內(nèi)存大小
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | using UnityEngine; using UnityEditor; using System.Reflection; public class TextureSizeExample : MonoBehaviour { ???? [SerializeField] ???? private Texture2D texture; ???? private void Start() ???? { ???????? long fileSize = GetTextureFileSize(texture); ???????? long memorySize = GetTextureRuntimeMemorySize(texture); ???????? Debug.Log( "Texture File Size: " + fileSize + " bytes" ); ???????? Debug.Log( "Texture Runtime Memory Size: " + memorySize + " bytes" ); ???? } ???? private static long GetTextureFileSize(Texture2D texture) ???? { ???????? long fileSize = 0; ???????? Type textureUtilType = typeof (TextureImporter).Assembly.GetType( "UnityEditor.TextureUtil" ); ???????? MethodInfo getStorageMemorySizeLongMethod = textureUtilType.GetMethod( "GetStorageMemorySizeLong" , BindingFlags.Static | BindingFlags.Public); ???????? fileSize = ( long )getStorageMemorySizeLongMethod.Invoke( null , new object [] { texture }); ???????? return fileSize; ???? } ???? private static long GetTextureRuntimeMemorySize(Texture2D texture) ???? { ???????? long memorySize = 0; ???????? Type textureUtilType = typeof (TextureImporter).Assembly.GetType( "UnityEditor.TextureUtil" ); ???????? MethodInfo getRuntimeMemorySizeLongMethod = textureUtilType.GetMethod( "GetRuntimeMemorySizeLong" , BindingFlags.Static | BindingFlags.Public); ???????? memorySize = ( long )getRuntimeMemorySizeLongMethod.Invoke( null , new object [] { texture }); ???????? return memorySize; ???? } } |
注意事項(xiàng)
- 確保在使用反射訪問UnityEditor.TextureUtil類之前,已經(jīng)導(dǎo)入了UnityEditor命名空間和System.Reflection命名空間。
- 使用反射時(shí),需要使用BindingFlags.Static | BindingFlags.Public來(lái)獲取靜態(tài)公共方法。
- 在示例代碼中,我們使用了Texture2D類型的變量來(lái)表示Texture,你可以根據(jù)實(shí)際情況修改代碼以適應(yīng)不同的Texture類型。
繼續(xù)閱讀
我的微信
微信掃一掃
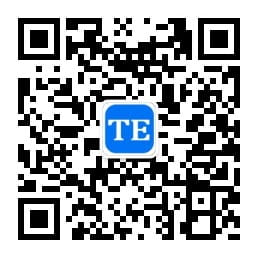
我的微信
惠生活福利社
微信掃一掃

我的公眾號(hào)
評(píng)論