這篇文章主要給大家介紹了利用Python實現斐波那契數列的幾種方法,文中通過示例代碼介紹的非常詳細,對大家的學習或者使用Python具有一定的參考學習價值,需要的朋友們下面來一起學習學習吧
文章源自四五設計網-http://www.wasochina.com/38773.html
斐波那契數列——經典例子,永不過時!!!文章源自四五設計網-http://www.wasochina.com/38773.html
1.for循環
1 2 3 4 5 6 7 8 | def fibonacci1(n): ???? a, b = 0 , 1 ???? for i in range (n): ???????? a, b = b, a + b ???????? print (a) fibonacci1( 3 ) |
或文章源自四五設計網-http://www.wasochina.com/38773.html
1 2 3 4 5 6 7 | def fib1(w): ???? a, b = 1 , 1 ???? for i in range (w - 1 ): ???????? a, b = b, a + b ???? return a ??????? print (fib1( 3 )) |
[^1]剛好得出這個位置的數文章源自四五設計網-http://www.wasochina.com/38773.html
2.while循環
1 2 3 4 5 6 7 8 9 | def fibnaqi2(m): ???? a, b = 0 , 1 ???? i = 0 ???? while i < m: ???????? print (b) ???????? a, b = b, a + b ???????? i + = 1 fibnaqi2( 4 ) |
[^1]剛好得出這個位置的數文章源自四五設計網-http://www.wasochina.com/38773.html
3.使用遞歸
1 2 3 4 5 6 | def fib2(q): ???? if q = = 1 or q = = 2 : ???????? return 1 ???? return fib2(q - 1 ) + fib2(q - 2 ) ??????? print (fib2( 9 )) |
4.遞歸+for循環
1 2 3 4 5 6 7 8 9 10 | def fibnacci3(p): ???? lst = [] ???? for i in range (p): ???????? if i = = 1 or i = = 0 : ???????????? lst.append( 1 ) ???????? else : ???????????? lst.append(lst[i - 1 ] + lst[i - 2 ]) ???? print (lst) fibnacci3( 5 ) |
5.遞歸+while循環
1 2 3 4 5 6 7 8 9 10 11 12 | def fibnacci4(k): ???? lis = [] ???? i = 0 ???? while i<k: ???????? if i = = 0 or i = = 1 : ???????????? lis.append( 1 ) ???????? else : ???????????? lis.append(lis[i - 2 ] + lis[i - 1 ]) ???????? i + = 1 ???? print (lis) fibnacci4( 6 ) |
6.遞歸+定義函數+for循環
1 2 3 4 5 6 7 8 9 10 | def fibnacci5(o): ???? def fn(i): ???????? if i < 2 : ???????????? return 1 ???????? else : ???????????? return (fn(i - 2 ) + fn(i - 1 )) ???? for i in range (o): ???????? print (fn(i)) ??????? fibnacci5( 8 ) |
7.指定列表
1 2 3 4 5 6 7 8 9 10 11 | def fib3(e): ???? if e = = 1 : ???????? return [ 1 ] ???? if e = = 2 : ???????? return [ 1 , 1 ] ???? fibs = [ 1 , 1 ] ???? for i in range ( 2 , e): ???????? fibs.append(fibs[ - 1 ] + fibs[ - 2 ]) ???? return fibs print (fib3( 12 )) |
趣方程求解
題目描述文章源自四五設計網-http://www.wasochina.com/38773.html
二次方程式 ax**2 + bx + c = 0 (a、b、c 用戶提供,為實數,a ≠ 0)文章源自四五設計網-http://www.wasochina.com/38773.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | # 導入 cmath(復雜數學運算) 模塊 import cmath a = float ( input ( '輸入 a: ' )) b = float ( input ( '輸入 b: ' )) c = float ( input ( '輸入 c: ' )) # 計算 d = (b * * 2 ) - ( 4 * a * c) # 兩種求解方式 sol1 = ( - b - cmath.sqrt(d)) / ( 2 * a) sol2 = ( - b + cmath.sqrt(d)) / ( 2 * a) print ( '結果為 {0} 和 {1}' . format (sol1, sol2)) |
pandas 每日一練
1 2 3 4 5 6 7 8 9 10 11 12 13 | # -*- coding = utf-8 -*- # @Time : 2022/7/26 21:48 # @Author : lxw_pro # @File : pandas -8 練習.py # @Software : PyCharm import pandas as pd import numpy as np df = pd.read_excel( 'text5.xlsx' ) print (df) print () |
程序運行結果如下:文章源自四五設計網-http://www.wasochina.com/38773.html
? ?Unnamed: 0 Unnamed: 0.1 ?project ?... ? ? ? ? ? test_time ? ? ? date ? ? ?time
0 ? ? ? ? ? 0 ? ? 00:00:00 ? Python ?... 2022-06-20 18:30:20 2022-06-20 ?18:30:20
1 ? ? ? ? ? 1 ? ? ? ? ? ?1 ? ? Java ?... 2022-06-18 19:40:20 2022-06-18 ?19:40:20
2 ? ? ? ? ? 2 ? ? ? ? ? ?2 ? ? ? ?C ?... 2022-06-08 13:33:20 2022-06-08 ?13:33:20
3 ? ? ? ? ? 3 ? ? ? ? ? ?3 ? ?MySQL ?... 2021-12-23 11:26:20 2021-12-23 ?11:26:20
4 ? ? ? ? ? 4 ? ? ? ? ? ?4 ? ?Linux ?... 2021-12-20 18:20:20 2021-12-20 ?18:20:20
5 ? ? ? ? ? 5 ? ? ? ? ? ?5 ? ? Math ?... 2022-07-20 16:30:20 2022-07-20 ?16:30:20
6 ? ? ? ? ? 6 ? ? ? ? ? ?6 ?English ?... 2022-06-23 15:30:20 2022-06-23 ?15:30:20
7 ? ? ? ? ? 7 ? ? ? ? ? ?7 ? Python ?... 2022-07-19 09:30:20 2022-07-19 ?09:30:20
[8 rows x 7 columns]文章源自四五設計網-http://www.wasochina.com/38773.html
41、將test_time列設置為索引文章源自四五設計網-http://www.wasochina.com/38773.html
1 2 3 | print (df.set_index( 'test_time' )) ??????? print () |
程序運行結果如下:
Unnamed: 0 Unnamed: 0.1 ?... ? ? ? date ? ? ?time
test_time ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ...
2022-06-20 18:30:20 ? ? ? ? ? 0 ? ? 00:00:00 ?... 2022-06-20 ?18:30:20
2022-06-18 19:40:20 ? ? ? ? ? 1 ? ? ? ? ? ?1 ?... 2022-06-18 ?19:40:20
2022-06-08 13:33:20 ? ? ? ? ? 2 ? ? ? ? ? ?2 ?... 2022-06-08 ?13:33:20
2021-12-23 11:26:20 ? ? ? ? ? 3 ? ? ? ? ? ?3 ?... 2021-12-23 ?11:26:20
2021-12-20 18:20:20 ? ? ? ? ? 4 ? ? ? ? ? ?4 ?... 2021-12-20 ?18:20:20
2022-07-20 16:30:20 ? ? ? ? ? 5 ? ? ? ? ? ?5 ?... 2022-07-20 ?16:30:20
2022-06-23 15:30:20 ? ? ? ? ? 6 ? ? ? ? ? ?6 ?... 2022-06-23 ?15:30:20
2022-07-19 09:30:20 ? ? ? ? ? 7 ? ? ? ? ? ?7 ?... 2022-07-19 ?09:30:20
[8 rows x 6 columns]
42、生成一個和df長度相同的隨機數dataframe
1 2 3 4 | df1 = pd.DataFrame(pd.Series(np.random.randint( 1 , 10 , 8 ))) print (df1) ??????? print () |
程序運行結果如下:
? ?0
0 ?1
1 ?3
2 ?2
3 ?7
4 ?7
5 ?3
6 ?5
7 ?1
43、將上一題生成的dataframe與df合并
1 2 3 4 | df = pd.concat([df, df1], axis = 1 ) print (df) ??????? print () |
程序運行結果如下:
? ?Unnamed: 0 Unnamed: 0.1 ?project ?... ? ? ? date ? ? ?time ?0
0 ? ? ? ? ? 0 ? ? 00:00:00 ? Python ?... 2022-06-20 ?18:30:20 ?1
1 ? ? ? ? ? 1 ? ? ? ? ? ?1 ? ? Java ?... 2022-06-18 ?19:40:20 ?3
2 ? ? ? ? ? 2 ? ? ? ? ? ?2 ? ? ? ?C ?... 2022-06-08 ?13:33:20 ?2
3 ? ? ? ? ? 3 ? ? ? ? ? ?3 ? ?MySQL ?... 2021-12-23 ?11:26:20 ?7
4 ? ? ? ? ? 4 ? ? ? ? ? ?4 ? ?Linux ?... 2021-12-20 ?18:20:20 ?7
5 ? ? ? ? ? 5 ? ? ? ? ? ?5 ? ? Math ?... 2022-07-20 ?16:30:20 ?3
6 ? ? ? ? ? 6 ? ? ? ? ? ?6 ?English ?... 2022-06-23 ?15:30:20 ?5
7 ? ? ? ? ? 7 ? ? ? ? ? ?7 ? Python ?... 2022-07-19 ?09:30:20 ?1
[8 rows x 8 columns]
44、生成新的一列new為popularity列減去之前生成隨機數列
1 2 3 4 | df[ 'new' ] = df[ 'popularity' ] - df[ 0 ] print (df) ??????? print () |
程序運行結果如下:
? Unnamed: 0 Unnamed: 0.1 ?project ?popularity ?... ? ? ? date ? ? ?time ?0 ?new
0 ? ? ? ? ? 0 ? ? 00:00:00 ? Python ? ? ? ? ?95 ?... 2022-06-20 ?18:30:20 ?1 ? 94
1 ? ? ? ? ? 1 ? ? ? ? ? ?1 ? ? Java ? ? ? ? ?92 ?... 2022-06-18 ?19:40:20 ?3 ? 89
2 ? ? ? ? ? 2 ? ? ? ? ? ?2 ? ? ? ?C ? ? ? ? 145 ?... 2022-06-08 ?13:33:20 ?2 ?143
3 ? ? ? ? ? 3 ? ? ? ? ? ?3 ? ?MySQL ? ? ? ? 141 ?... 2021-12-23 ?11:26:20 ?7 ?134
4 ? ? ? ? ? 4 ? ? ? ? ? ?4 ? ?Linux ? ? ? ? ?84 ?... 2021-12-20 ?18:20:20 ?7 ? 77
5 ? ? ? ? ? 5 ? ? ? ? ? ?5 ? ? Math ? ? ? ? 148 ?... 2022-07-20 ?16:30:20 ?3 ?145
6 ? ? ? ? ? 6 ? ? ? ? ? ?6 ?English ? ? ? ? 146 ?... 2022-06-23 ?15:30:20 ?5 ?141
7 ? ? ? ? ? 7 ? ? ? ? ? ?7 ? Python ? ? ? ? 149 ?... 2022-07-19 ?09:30:20 ?1 ?148
[8 rows x 9 columns]
45、檢查數據中是否含有任何缺失值
1 2 3 4 | jch = df.isnull().values. any () print (jch)??? # 運行結果為:False ??????? print () |
46、將popularity列類型轉換為浮點數
1 2 3 4 | fds = df[ 'popularity' ].astype(np.float64) print (fds) ??????? print () |
程序運行結果如下:
0 ? ? 95.0
1 ? ? 92.0
2 ? ?145.0
3 ? ?141.0
4 ? ? 84.0
5 ? ?148.0
6 ? ?146.0
7 ? ?149.0
Name: popularity, dtype: float64
47、計算popularity大于100的次數
1 2 3 4 | cs = len (df[df[ 'popularity' ] > 100 ]) print (cs)??? # 運行結果為:5 ??????? print () |
48、查看project列共有幾種學歷
1 2 3 4 | ckj = df[ 'project' ].nunique() print (ckj)??? # 運行結果為:7 ??????? print () |
49、查看每科出現的次數
1 2 3 4 | ckc = df.project.value_counts() print (ckc) print () |
程序運行結果如下:
Python ? ? 2
Java ? ? ? 1
C ? ? ? ? ?1
MySQL ? ? ?1
Linux ? ? ?1
Math ? ? ? 1
English ? ?1
Name: project, dtype: int64
50、提取popularity與new列的和大于136的最后3行
1 2 3 4 | df1 = df[[ 'popularity' , 'new' ]] hh = df1. apply (np. sum , axis = 1 ) res = df.iloc[np.where(hh > 136 )[ 0 ][ - 3 :], :] print (res) |
程序運行結果如下:
? ?Unnamed: 0 Unnamed: 0.1 ?project ?popularity ?... ? ? ? date ? ? ?time ?0 ?new
5 ? ? ? ? ? 5 ? ? ? ? ? ?5 ? ? Math ? ? ? ? 148 ?... 2022-07-20 ?16:30:20 ?3 ?145
6 ? ? ? ? ? 6 ? ? ? ? ? ?6 ?English ? ? ? ? 146 ?... 2022-06-23 ?15:30:20 ?5 ?141
7 ? ? ? ? ? 7 ? ? ? ? ? ?7 ? Python ? ? ? ? 149 ?... 2022-07-19 ?09:30:20 ?1 ?148
[3 rows x 9 columns]
到此這篇關于Python實現斐波那契數列的多種寫法總結的文章就介紹到這了
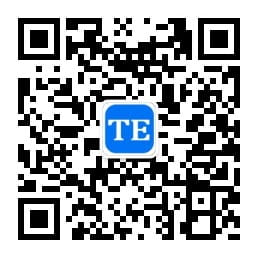

評論